mirror of
https://codeberg.org/forgejo/forgejo
synced 2024-05-05 03:12:54 +02:00
Fixes https://github.com/go-gitea/gitea/issues/25094 `GetBlobContent` will only get the first 1024 bytes, if the README.md size is larger than 1024 bytes, We can not render the rest of them. After this fix, we should provide the limited size to read when call `GetBlobContent`. After: 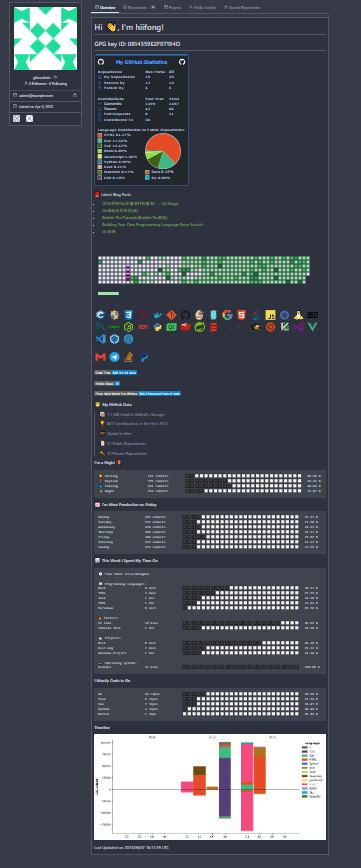 --------- Co-authored-by: wxiaoguang <wxiaoguang@gmail.com>
103 lines
2.2 KiB
Go
103 lines
2.2 KiB
Go
// Copyright 2015 The Gogs Authors. All rights reserved.
|
|
// Copyright 2019 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package git
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/base64"
|
|
"io"
|
|
|
|
"code.gitea.io/gitea/modules/typesniffer"
|
|
"code.gitea.io/gitea/modules/util"
|
|
)
|
|
|
|
// This file contains common functions between the gogit and !gogit variants for git Blobs
|
|
|
|
// Name returns name of the tree entry this blob object was created from (or empty string)
|
|
func (b *Blob) Name() string {
|
|
return b.name
|
|
}
|
|
|
|
// GetBlobContent Gets the limited content of the blob as raw text
|
|
func (b *Blob) GetBlobContent(limit int64) (string, error) {
|
|
if limit <= 0 {
|
|
return "", nil
|
|
}
|
|
dataRc, err := b.DataAsync()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
defer dataRc.Close()
|
|
buf, err := util.ReadWithLimit(dataRc, int(limit))
|
|
return string(buf), err
|
|
}
|
|
|
|
// GetBlobLineCount gets line count of the blob
|
|
func (b *Blob) GetBlobLineCount() (int, error) {
|
|
reader, err := b.DataAsync()
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
defer reader.Close()
|
|
buf := make([]byte, 32*1024)
|
|
count := 1
|
|
lineSep := []byte{'\n'}
|
|
|
|
c, err := reader.Read(buf)
|
|
if c == 0 && err == io.EOF {
|
|
return 0, nil
|
|
}
|
|
for {
|
|
count += bytes.Count(buf[:c], lineSep)
|
|
switch {
|
|
case err == io.EOF:
|
|
return count, nil
|
|
case err != nil:
|
|
return count, err
|
|
}
|
|
c, err = reader.Read(buf)
|
|
}
|
|
}
|
|
|
|
// GetBlobContentBase64 Reads the content of the blob with a base64 encode and returns the encoded string
|
|
func (b *Blob) GetBlobContentBase64() (string, error) {
|
|
dataRc, err := b.DataAsync()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
defer dataRc.Close()
|
|
|
|
pr, pw := io.Pipe()
|
|
encoder := base64.NewEncoder(base64.StdEncoding, pw)
|
|
|
|
go func() {
|
|
_, err := io.Copy(encoder, dataRc)
|
|
_ = encoder.Close()
|
|
|
|
if err != nil {
|
|
_ = pw.CloseWithError(err)
|
|
} else {
|
|
_ = pw.Close()
|
|
}
|
|
}()
|
|
|
|
out, err := io.ReadAll(pr)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return string(out), nil
|
|
}
|
|
|
|
// GuessContentType guesses the content type of the blob.
|
|
func (b *Blob) GuessContentType() (typesniffer.SniffedType, error) {
|
|
r, err := b.DataAsync()
|
|
if err != nil {
|
|
return typesniffer.SniffedType{}, err
|
|
}
|
|
defer r.Close()
|
|
|
|
return typesniffer.DetectContentTypeFromReader(r)
|
|
}
|