mirror of
https://codeberg.org/forgejo/forgejo
synced 2024-05-08 12:52:10 +02:00
1. Add `<overflow-menu>` web component 2. Rename `<gitea-origin-url>` to `<origin-url>` and make filenames match. <img width="439" alt="image" src="https://github.com/go-gitea/gitea/assets/115237/2fbe4ca4-110b-4ad2-8e17-c1e116ccbd74"> <img width="444" alt="Screenshot 2024-03-02 at 21 36 52" src="https://github.com/go-gitea/gitea/assets/115237/aa8f786e-dc8c-4030-b12d-7cfb74bdfd6e"> <img width="537" alt="Screenshot 2024-03-03 at 03 05 06" src="https://github.com/go-gitea/gitea/assets/115237/fddd50aa-adf1-4b4b-bd7f-caf30c7b2245"> 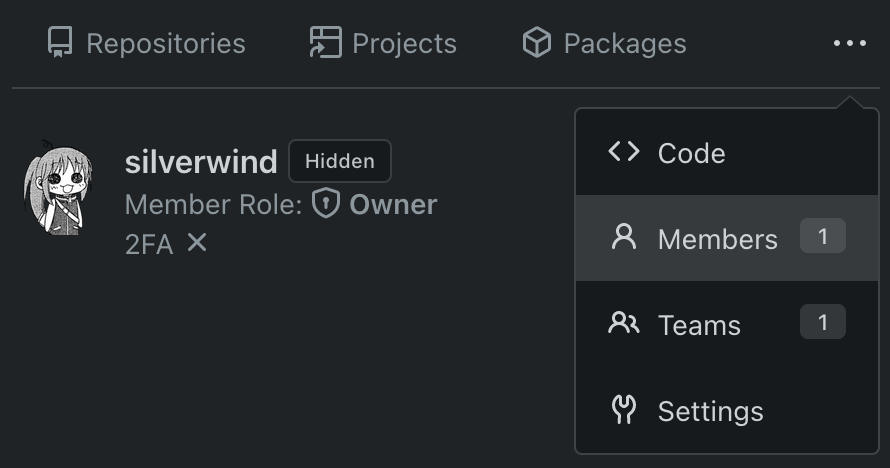 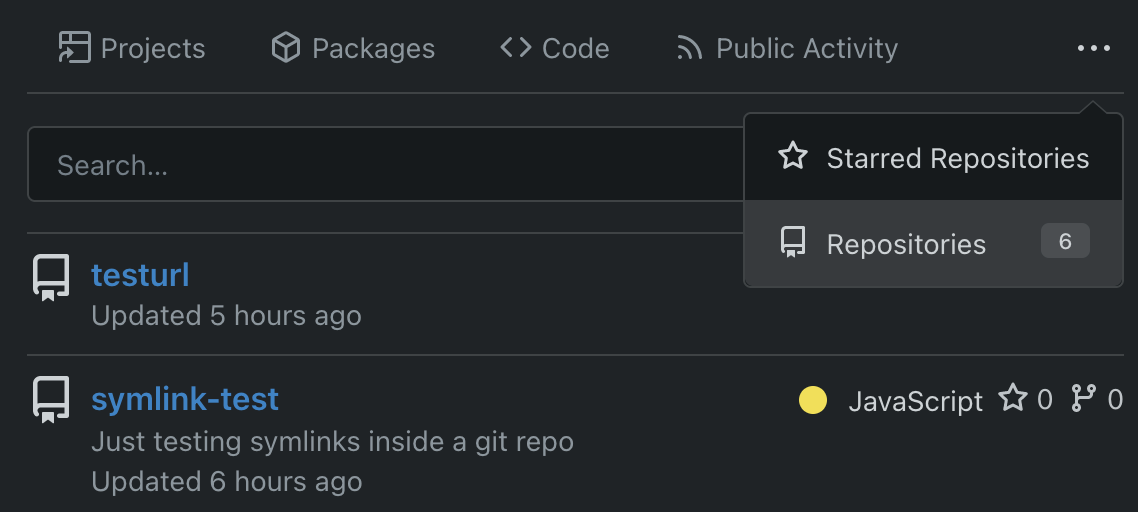 TODO: - [x] Check if removal of `requestAnimationFrame` is possible to avoid flash of content. Likely needs a `MutationObserver`. - [x] Hide tippy when button is removed from DOM. - [x] ~~Implement right-aligned items (https://github.com/go-gitea/gitea/pull/28976)~~. Not going to do it. - [x] Clean up CSS so base element has no background and add background via tailwind instead. - [x] Use it for org and user page. --------- Co-authored-by: Giteabot <teabot@gitea.io> Co-authored-by: wxiaoguang <wxiaoguang@gmail.com> (cherry picked from commit 256a1eeb9a67b18c62a10f5909b584b7b220848a) Conflicts: options/locale/locale_en-US.ini templates/package/content/cargo.tmpl templates/package/content/cran.tmpl templates/package/content/debian.tmpl templates/package/content/maven.tmpl
48 lines
2.1 KiB
Go
48 lines
2.1 KiB
Go
// Copyright 2023 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package timeutil
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"code.gitea.io/gitea/modules/setting"
|
|
"code.gitea.io/gitea/modules/test"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestDateTime(t *testing.T) {
|
|
testTz, _ := time.LoadLocation("America/New_York")
|
|
defer test.MockVariableValue(&setting.DefaultUILocation, testTz)()
|
|
|
|
refTimeStr := "2018-01-01T00:00:00Z"
|
|
refDateStr := "2018-01-01"
|
|
refTime, _ := time.Parse(time.RFC3339, refTimeStr)
|
|
refTimeStamp := TimeStamp(refTime.Unix())
|
|
|
|
assert.EqualValues(t, "-", DateTime("short", nil))
|
|
assert.EqualValues(t, "-", DateTime("short", 0))
|
|
assert.EqualValues(t, "-", DateTime("short", time.Time{}))
|
|
assert.EqualValues(t, "-", DateTime("short", TimeStamp(0)))
|
|
|
|
actual := DateTime("short", "invalid")
|
|
assert.EqualValues(t, `<absolute-date weekday="" year="numeric" month="short" day="numeric" date="invalid">invalid</absolute-date>`, actual)
|
|
|
|
actual = DateTime("short", refTimeStr)
|
|
assert.EqualValues(t, `<absolute-date weekday="" year="numeric" month="short" day="numeric" date="2018-01-01T00:00:00Z">2018-01-01T00:00:00Z</absolute-date>`, actual)
|
|
|
|
actual = DateTime("short", refTime)
|
|
assert.EqualValues(t, `<absolute-date weekday="" year="numeric" month="short" day="numeric" date="2018-01-01T00:00:00Z">2018-01-01</absolute-date>`, actual)
|
|
|
|
actual = DateTime("short", refDateStr)
|
|
assert.EqualValues(t, `<absolute-date weekday="" year="numeric" month="short" day="numeric" date="2018-01-01">2018-01-01</absolute-date>`, actual)
|
|
|
|
actual = DateTime("short", refTimeStamp)
|
|
assert.EqualValues(t, `<absolute-date weekday="" year="numeric" month="short" day="numeric" date="2017-12-31T19:00:00-05:00">2017-12-31</absolute-date>`, actual)
|
|
|
|
actual = DateTime("full", refTimeStamp)
|
|
assert.EqualValues(t, `<relative-time weekday="" year="numeric" format="datetime" month="short" day="numeric" hour="numeric" minute="numeric" second="numeric" data-tooltip-content data-tooltip-interactive="true" datetime="2017-12-31T19:00:00-05:00">2017-12-31 19:00:00 -05:00</relative-time>`, actual)
|
|
}
|