mirror of
https://codeberg.org/forgejo/forgejo
synced 2024-05-18 02:00:15 +02:00
1. Fix multiple error display for math and mermaid: 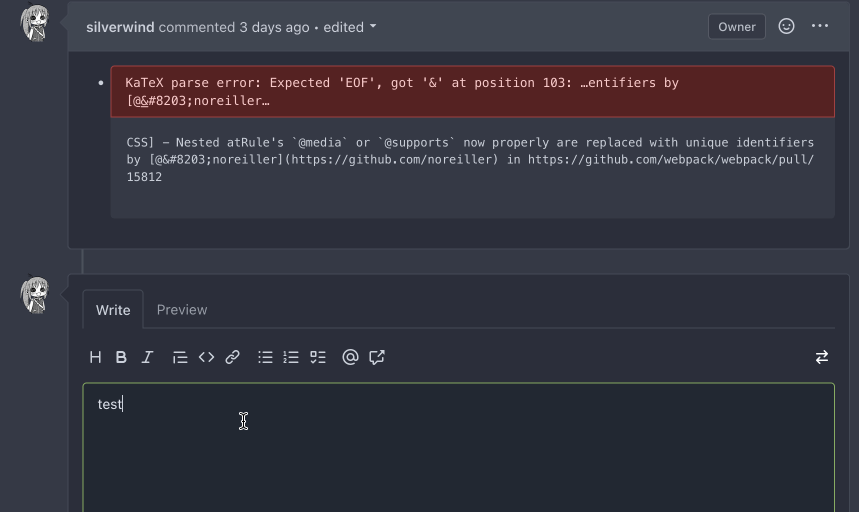 2. Fix height calculation of certain mermaid diagrams by reading the iframe inner height from it's document instead of parsing it from SVG: Before: <img width="866" alt="Screenshot 2023-04-11 at 11 56 27" src="https://user-images.githubusercontent.com/115237/231126480-b194e02b-ea8c-4ddf-8c79-50c525815d92.png"> After: <img width="855" alt="Screenshot 2023-04-11 at 11 56 35" src="https://user-images.githubusercontent.com/115237/231126494-5fe86a48-8d21-455a-8b95-79b6ee27a16f.png"> 3. Refactor error handling to a common function 4. Rename to `renderAsciicast` for consistency 5. Improve mermaid loading sequence Note: I did try `securityLevel: 'sandbox'` to make mermaid output a iframe directly, but that showed a bug in mermaid where the iframe style height was set incorrectly. Opened https://github.com/mermaid-js/mermaid/issues/4289 for this. --------- Co-authored-by: Giteabot <teabot@gitea.io>
38 lines
1.1 KiB
JavaScript
38 lines
1.1 KiB
JavaScript
import {displayError} from './common.js';
|
|
|
|
function targetElement(el) {
|
|
// The target element is either the current element if it has the
|
|
// `is-loading` class or the pre that contains it
|
|
return el.classList.contains('is-loading') ? el : el.closest('pre');
|
|
}
|
|
|
|
export async function renderMath() {
|
|
const els = document.querySelectorAll('.markup code.language-math');
|
|
if (!els.length) return;
|
|
|
|
const [{default: katex}] = await Promise.all([
|
|
import(/* webpackChunkName: "katex" */'katex'),
|
|
import(/* webpackChunkName: "katex" */'katex/dist/katex.css'),
|
|
]);
|
|
|
|
for (const el of els) {
|
|
const target = targetElement(el);
|
|
if (target.hasAttribute('data-render-done')) continue;
|
|
const source = el.textContent;
|
|
const displayMode = el.classList.contains('display');
|
|
const nodeName = displayMode ? 'p' : 'span';
|
|
|
|
try {
|
|
const tempEl = document.createElement(nodeName);
|
|
katex.render(source, tempEl, {
|
|
maxSize: 25,
|
|
maxExpand: 50,
|
|
displayMode,
|
|
});
|
|
target.replaceWith(tempEl);
|
|
} catch (error) {
|
|
displayError(target, error);
|
|
}
|
|
}
|
|
}
|